Software development projects tend to be very complicated and complex, which is why tools that help you with various tasks are so useful. Terraform – created by a company called HashiCorp – is one such solution. In this Terraform tutorial I’ll show tell you all about this technology, and show you how to use it together with AWS.
Terraform is an infrastructure as code tool that can help you with provisioning your infrastructure in the cloud, and I’m somewhat surprised it didn’t find widespread adoption yet. In the latest Stack Overflow Developer Survey, only 12,3% of professional devs named it as one of their fundamental tools.
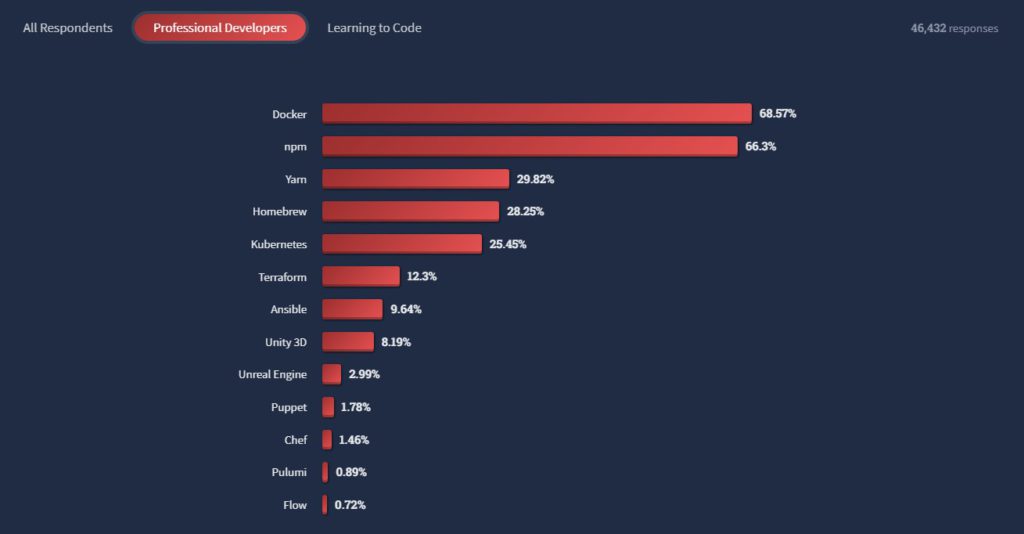
Who is this technology worth using? How can it help you? How to performa a basic Terraform-AWS integration? Read this Terraform tutorial to find out.
What is Terraform?
Let’s start with the basics. Terraform is an infrastructure as code tool you can use to define resources in readable configuration files – both in the cloud and on-premise. This allows you to create an optimized and consistent workflow and manage various resources – both high-level (SaaS features, DNS entries), and low-level (storage, network, compute power).
Terraform use cases
You can use Terraform for several things, such as provisioning of cloud resources, multi-cloud deployment, creating self-service clusters, infrastructure deployment and scaling.
Terraform pricing and editions
There are three main editions of Terraform:
- Terraform Open Source – the basic version that you interact with using the command line. You can use it with any cloud provider to provision thousands of types of services and resources. Terraform Open Source is, as the name suggests, completely free to use.
- Terraform Cloud – the paid variant of Terraform, which is available in the SaaS model (Software as a Service). The tool runs in a remote environment or a local CLI, and you can easily integrate it with standard version control systems, i.e. GitHub, BitBucket, and GitLab. It also offers a richer, user-friendly interface that you can use to interact with its various features and functions. The price starts at $20 per user, but, to make things a bit more confusing, there’s also a free version of Terraform Cloud with a limited feature set.
- Terraform Enterprise / Business – the version meant for enterprise-grade companies. It allows you to set up your own (self-hosted) distribution of Terraform Cloud. The resource limits are customizable, and so is the pricing – you’ll have to contact the company directly to get more details.
Terraform advantages and disadvantages
There are a number of distinct advantages Terraform offers developers. Here are some I consider to be the most important:
- You save a lot of time – Terraform manages some things automatically using default values. For example: as we can see in our example below, when we configure aws_security_group, there is no need to add vpi_id. Terreform will default the region’s default VPC. You can also create Terraform modules – self-contained configuration packages.
- Cooperation is easier – you can add a Git repo to the configuration so that your entire team uses the same scripts when working on Terraform projects.
- Great compatibility with AWS – Terraform creators are in the AWS Network program, which ensures very good compatibility with Amazon Web Services.
When it comes to disadvantages, there’s really only one thing, directly correlated with Terraform’s biggest boon. As I’ve mentioned, the tool does some things automatically using default variables, which helps save a lot of time. However, in some cases, and to some developers, these default settings might be too limiting. It can be an issue, but as far as I’m concerned, it’s still better than doing everything manually.
Terraform functions
Terraform functions help you complete various tasks. The tool uses nine different types of them:
- Type Conversion – you use them to convert the data type of the variable. Example: nonsensitive.
- String – functions that have to do with String manipulation, e.g. the join function.
- IP Network – network-related functions, e.g. cidrhost.
- Collection – you use this type of function to validate keys and values, as well as sort data. Example: the splat operator.
- Encoding – these help you with encoding and decoding data, e.g. textencodebase64.
- Hash and Crypto – used for anonymizing and encrypting data, e.g. filesha256.
- Date and Time – you use them to manipulate or alter time and dates. Example: timeadd.
- Numeric – used to identify and manage numeric values, e.g. parseint.
- File System – you use this for file manipulation in a Terraform directory. Example: templatefile.
How to install Terraform?
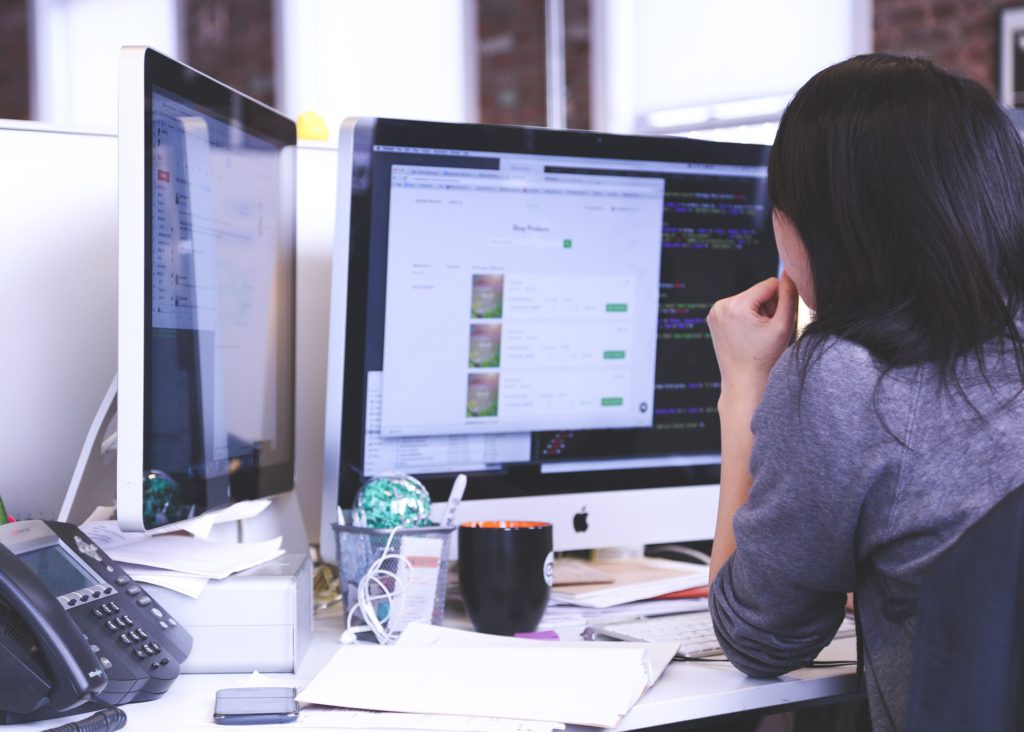
Terraform installation is very easy. The tool supports Windows, Linux, FreeBSD, OpenBSD, Solaris, and macOS operating systems. To install it simply download the appropriate Terraform binary package from the official website, and run it in a manner typical for your OS. In the case of some systems, you can also use a package manager.
Terraform & AWS – how to use them together
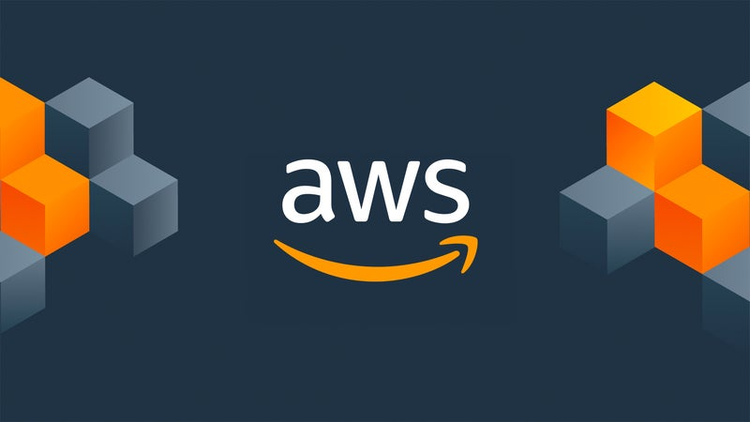
Yes, we know that AWS has its own cloud formation. However, it requires you to write a lot of text. If you use Terraform, you can do the same thing much faster, and have a piece of infrastructure ready in no time. In this part of the article, I’ll show you how to do this, step-by-step.
Disclaimer: I’ve used the Open Source version of Terraform for the purposes of this tutorial.
1. Adding a provider
The first thing to do is configure a provider. To do this, you just need to add two objects. The first object will inform Terraform that you want to use AWS for your project.
terraform { required_providers { aws = { source = "hashicorp/aws" version = "~> 4.0" } } } # Configure the AWS Provider provider "aws" { region = "us-east-1" }
The second object will be the configuration itself since you need to configure the Terraform provider to connect to AWS.
provider "aws" { region = "us-west-2" access_key = "my-access-key" secret_key = "my-secret-key" }
Please note, that this is just an example. It’s not recommended to add credentials like that. To do things right you’ll need to use one of the following options:
- Environmental variables – we can use exported variables.
export AWS_ACCESS_KEY_ID="key_id" export AWS_SECRET_ACCESS_KEY="secret_ket" export AWS_REGION="eu-west-1" and then in the script: provider "aws" {}
- Shared configuration files – we can use configuration stored in external files. By default they are atored in your home director in .aws folder. If you do not specify profile, the default will be used
provider "aws" { shared_config_files = ["/Users/{your_user}/.aws/conf"] shared_credentials_files = ["/Users/{your_user}/.aws/creds"] profile = "customprofile" }
- IAM Role – we can use AWS Role ARN to supply the credentials.
provider "aws" { assume_role { role_arn = "arn:aws:iam::12256459012:role/role_name" session_name = "session_name" external_id = "external_id" } }
- IAR Role and a token – same like before but with token.
provider "aws" { assume_role_with_web_identity { role_arn = "arn:aws:iam::12256459012:role/role_name" session_name = "session_name" web_identity_token_file = "/Users/{your_user}/aws/security-token" } }
- AWS Profile – we can use aws credential process. We just need to configure it for our profile. To configure it see AWS documentation.
provider "aws" { profile = "your_profile" }
2. Provisioning the infrastructure
Now, it’s time to provision the infrastrutcure. First, we can start with a simple example using one EC2 with a simple web server. You need to do the following things:
- You need to use previously configured Terraform providers.
- You have to configure a Security group. This is required to open the traffic from the Internet. Without this, Terraform would add a default security group.
- You need to have a configuration of the EC2 instance. You’ll have to add your new SG to the configuration.
- At user_date, you can add some configuration scripts, etc. You are essentially creating a dummy web page.
- The last thing is the output object with the public IP that you can use to enter.
And here’s the exact code fragment that’ll get you there:
# Configure the AWS provider provider "aws" { region = "eu-west-1" } # Create a Security Group for an EC2 instance resource "aws_security_group" "instance" { name = "terraform-example-instance" ingress { from_port = 8080 to_port = 8080 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } } # Create an EC2 instance resource "aws_instance" "example" { ami = "ami-785db401" instance_type = "t2.micro" vpc_security_group_ids = ["${aws_security_group.instance.id}"] user_data = <<-EOF #!/bin/bash echo "Hello, World" > index.html nohup busybox httpd -f -p 8080 & EOF tags { Name = "terraform-example" } } # Output variable: Public IP address output "public_ip" { value = "${aws_instance.example.public_ip}" }
3. Applying Terraform
Now that you have your Terraform configuration file, it’s time to apply it so that it’s successfully initialized. To do this, you’ll need to enter a couple of Terraform commands:
- terraform init command – initializing provider plugins;
- terraform validate command – validating the file;
- terraform plan command – it’ll let you see all the changes that you want to apply;
- terraform apply command – executing the configuration.
Also, If you want to clean up all the configuration, you just need to use the terraform destroy command.
4. Connecting Terraform with GIT
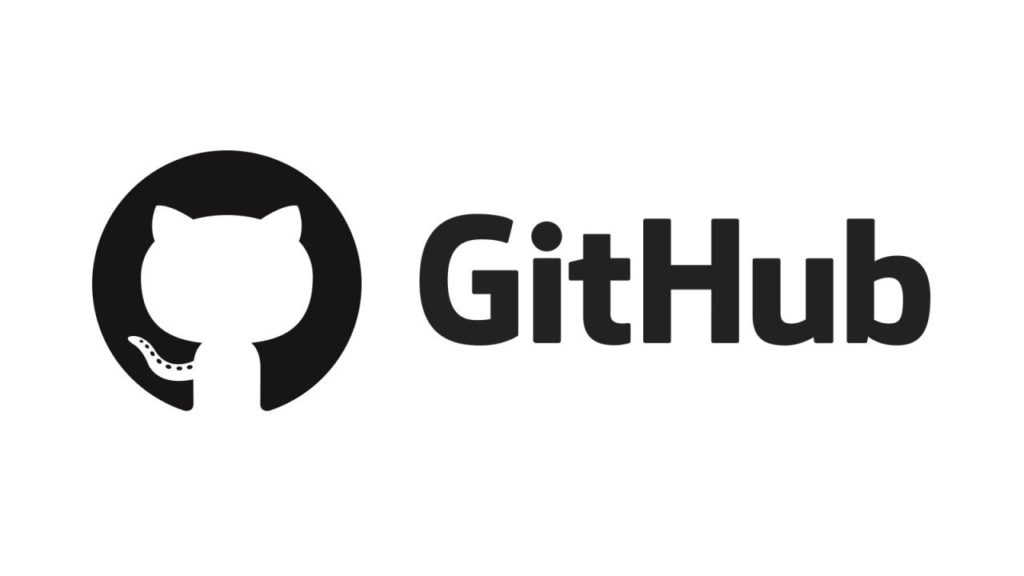
Cooperation between a couple of developers on the configuration of one infrastructure can be very tricky. There’s one thing you can do to ensure that everyone works on the newest version – you can connect Terraform with Git. It’ll store the latest Terraform state, which can be very advantageous. To do this, you simply have to add one object by using the following code fragment:
# Define Terraform backend using an S3 bucket for storing the Terraform state terraform { backend "s3" { bucket = "terraform-state" key = "terraform-state/terraform.tfstate" region = "eu-west-1" } }
And that’s it! Terraform should now be ready to work with your AWS-based cloud infrastructure. Quite easy, wasn’t it? You can also read yet another instruction on configuring Terraform and AWS on Semaphore’s blog.
Conclusion
As you can see, Terraform is a pretty useful tool that can make the work on infrastructure substantially quicker. Terraform management and configuration is also very easy, as long as you know what you’re doing or are willing to learn. I’ve used it in my work, and it allowed me to create a small infrastructure for a simple web server in just one day, which is probably the best recommendation I can give.
If you have any questions or problems with importing your existing resources, HashiCorp provided very comprehensive Terraform documentation – you’ll likely find what you’re looking for there.
Are you looking for experienced software developers?
And if you’re looking for someone who can help you create a system with a well-planned infrastructure, Pretius has a great deal of experience with various technologies and industries. We’ll likely know how to meet your requirements. Write us at hello@pretius.com or use the contact form below – we’ll get back to you in 48 hours.
Terraform tutorial FAQ
Here are answers to some standard Terraform questions.
Is Terraform free?
Yes, there is a free version of Terraform – Terraform Open Source. There’s also a free variant of the paid Terraform Cloud product, called Terraform Free.
What is the Terraform’s code?
Terraform is written in HashCorp’s own Configuration Language (HCL).
How does importing existing resources to Terraform work?
You can import resources and infrastructure using a CLI Terraform import command which tells the tool to read real-world infrastructure and update the state.
What is Terraform registry?
Terraform registry is an online directory in which you can browse different providers, modules, policy libraries, and run tasks.